Basic language principles#
This is a simple introduction to python for those who know nothing or very little about it. It starts with some practical exercise to give you a feeling for the language. Finally, it discusses more general concepts.
Exercise (1)
Before we dive into the details, let’s get our hands dirty!
Read the code below (don’t worry about 2 first lines).
Do you know what the code does?
import matplotlib.pyplot as plt
t = [0, 1, 2, 3, 4, 5]
s = [0, 1, 4, 9, 16, 25]
plt.plot(t, s, "o")
plt.show()
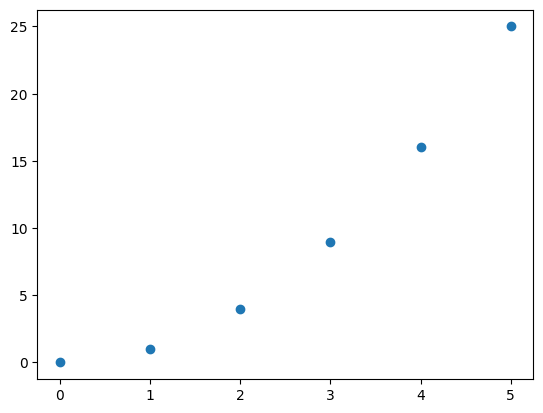
Exercise (2)
Now execute the code (Shift+Enter
).
Does it do what you expected?.
Play a little bit with the plot properties to, e.g., change style, color, etc.
First of all try to remove 'o'
from the plot
function and see what happens.
And then try adding 'r-'
in the same place where we had 'o'
before.
The plot
function has many options, and they can be accessed by pressing Shift+tab+tab
when cursor is pointing on the function (between parentheses) or simply checking the on-line help.
You can try out the following properties color='green', linestyle='dashed', marker='o', markerfacecolor='blue', markersize=12
.
Python principles#
Python is an interpreted language, meaning there is no explicit compilation step (same as Matlab or Mathematica for example)
The basic types build into Python include:
int
variable length integers, (e.g.x = -1234567890
)float
double precision floating point numbers, (e.g.x = 2.0
)complex
composed of two floats for real and imag part, (e.g.x = complex(1,5)
)str
unicode character strings, (e.g.x = 'This is a string'
, or equivalentlyx = "This is a string"
)bool
boolean which can be only True or False (e.g.x = True
)
The operations are supported as long as it makes sense for that type. For example, there is no division for strings. Addition
+
is defined and it results in the concatenation of the two strings.
Symbol |
Task Performed |
---|---|
+ |
Addition |
- |
Subtraction |
* |
Multiplication |
/ |
Floating point division |
+= |
In-place addition |
-= |
In-place subtraction |
*= |
In-place multiplication |
/= |
In-place floating point division |
// |
Floor division |
% |
Modulus or rest |
** or pow(a, b) |
Power |
abs(a) |
absolute value |
round(a) |
Banker’s rounding |
Python also accepts relational operators (note the difference,
==
means equality test and=
is an assignment)
Symbol |
Task Performed |
---|---|
== |
True, if it is equal |
!= |
True, if not equal to |
< |
less than |
> |
greater than |
<= |
less than or equal to |
>= |
greater than or equal to |
|
|
not |
negate a |
is |
True, if both are the same |
and |
True if both are True |
or |
True if any are are True |
^ |
True if one or the other but not both are True |
|
|
^ |
bitwise xor operator on |
& |
bitwise and operator on |
| |
bitwise or operator on |
>> |
right shift bitwise operation on |
<< |
left shift bitwise operation on |
Variable assignment is simpler than in other languages as you do not have to declare the type, and moreover, it can be changed during execution, e.g.
# Here x is initialized to 5 and Python then treats this as an integer
x = 5
# It can be incremented and have all the expected operations applied to it
x += 1
# Later on it can be used for something else e.g. string
x = "a string"
Comments are signified by the
#
symbol.
Exercise (3)
Using the variable assignment code shown above, first initialize a variable to 2
and then increment it by 2.
Then assign to the variable x the string value "my string"
.
# YOUR CODE HERE
Exercise (4)
What happens if you now add 5 to x?
# YOUR CODE HERE
You are most likely seeing the error similar to one below:
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-6-0550634c50dd> in <module>
2 x += 2
3 x = "my string"
----> 4 x + 5
5 x
TypeError: can only concatenate str (not "int") to str
This basically means that you cannot add integer value to a string. Do you understand why this has happened?